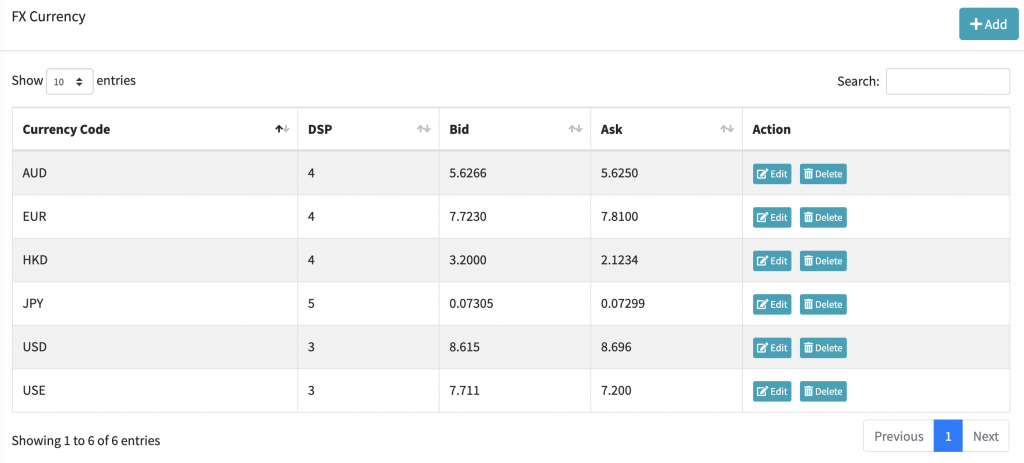
Prepare the view with table and javascript
var fx_currency = $('#fx_currency_table').DataTable({
processing: true,
serverSide: true,
bPaginate: true,
buttons:[],
ajax: '/fxcurrency',
columnDefs: [ {
"targets": 4,
"orderable": false,
"searchable": false
} ]
});
<div class="card-body">
<table class="table table-border table-striped" id="fx_currency_table" width="100%>
<thread>
<tr>
<th>Currency Code</th>
<th>DSP</th>
<th>Bid</th>
<th>Ask</th>
<th>@lang('message.action')</th>
</tr>
</thread>
</table>
</div>
Laravel Controller
public function index()
{
if (!auth()->user()->can('user.view') && !auth()->user()->can('user.create')) {
abort(403, 'Unauthorized action.');
}
if (request()->ajax()) {
$business_id = request()->session()->get('user.business_id');
$fxcurrency = FxCurrency::where('business_id', $business_id)
->select(['currency_code', 'id', 'dsp', 'ask', 'bid']);
return Datatables::of($fxcurrency)
->addColumn(
'action',
'
<button data-href="{{action(\'FxCurrencyController@edit\', [$id])}}" class="btn btn-xs btn-info btn-modal" data-container=".fxcurrency_edit_modal"><i class="fas fa-edit"></i> Edit</button>
<button data-href="{{action(\'FxCurrencyController@destroy\', [$id])}}" class="btn btn-xs btn-modal btn-info delete_user_button" data-container=".location_edit_modal"><i class="fas fa-trash-alt"></i> Delete</button>
‘
)
->removeColumn('id')
->rawColumns([4])
->editColumn('ask', function($fxcurrency) {
return $this->util->pack_dsp($fxcurrency->ask, $fxcurrency->dsp);
})
->editColumn('bid', function($fxcurrency) {
return $this->util->pack_dsp($fxcurrency->bid, $fxcurrency->dsp);
})
->make(false)
;
}
return view('fx_currency.index');
}